Debounce time is one of the most important and commonly used operators available from rxjs to developers when it comes to building efficient and effective applications in Angular. In this article, we'll discuss how you can learn debounce time operators work and how they should be used in Angular.
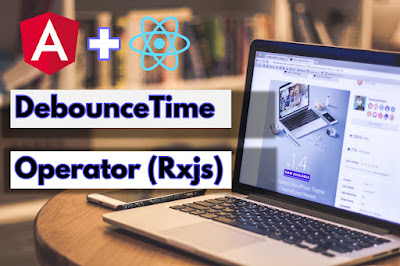 |
DebounceTime in angular |
We'll also look at debounce time example of where they can be used to get maximum benefit.
Reactive programming is an incredibly powerful tool when developing modern web applications. By using debounce time operators, developers can create user experiences that are smoother and more responsive than ever before.
It is important to stay up-to-date on the most efficient and effective ways to build applications. To this end, incorporating debounce time RxJS operators into an Angular project can be a great way to optimize code performance as it allows for better control over how often some events will fire.
What is Debounce Time Operator
The Debounce time operator takes an integer argument which defines a time window in milliseconds, during which it will ignore any incoming values from the source observable. This means that any new values received within this defined time window will be ignored until after the specified amount of time has passed.
Once that period has elapsed, only then will an emitted value be accepted and processed further down the line. This helps prevent any potential issues with multiple events firing at once while also helping maintain performance as well as accuracy of data handling across an entire application’s event stream.
Problem before Debounce time operator came
Before debounce time operator , we did not have the ability to control these kind of problems , at some point this is fine but what if you got to make an API call with the search input text , You are not supposed to make the API call everytime a character enters.
To avoid these unnecessary emissions, Rxjs introduced Debounce Time.
DebounceTime operator gives us the ability to limit how often a particular action can be performed within a given time frame. This helps us reduce the amount of unnecessary processing or requests made by our application, ultimately improving its performance and response time.
After using the Debounce time operator
We gave 1000 milliseconds interval for the emission. that's how it is reacting you can see the results:
This is how we can avoid having unnecessary emissions of an observable in our functionality logic.
How to use debounce time operator ?
You can use Debounce time operator in various places where you need some time to proceed the emission of an observable further.
Optimize Search Input for API results from server
When using this debounceTime operator, developers can set a time interval after which emissions are allowed. This can be used to ensure that frequent and repetitive API calls or requests are avoided, thus improving performance and reducing overhead on the server side.
Using the formControl from
reactive forms you can easily get the value entered by user and make operation on it as formControl is of observable type and you can use
valueChanges property to handle and make changes in the user input using Debounce time.
This is one of the dounce time example in angular, you can use this in other various places.
searchCntrl: FormControl();
apiResponse: any = null;
constructor() {
this.searchCntrl.valueChanges.pipe(
// Time in milliseconds between key events
, debounceTime(1000)
// subscription for response
).subscribe((text: string) => {
this.someApiCall(text).subscribe((res) => {
console.log('res', res);
this.apiResponse = res;
}, (err) => {
console.log('error', err);
});
});
}
The debounce Time also helps minimize redundant calls made by users when entering text into input fields on forms or search boxes by delaying such actions until after a certain amount of time has passed since their last action.
In addition , we can also avoid having unnecessary emissions of an observable where the user enters the same text again and again in the given period of that debounce time but we can avoid this problem too.
searchCntrl: FormControl();
apiResponse: any = null;
constructor() {
this.searchCntrl.valueChanges.pipe(
// Time in milliseconds between key events
, debounceTime(1000),
// added distinctUntilChanged here
distinctUntilChanged()
// subscription for response
).subscribe((text: string) => {
this.someApiCall(text).subscribe((res) => {
console.log('res', res);
this.apiResponse = res;
}, (err) => {
console.log('error', err);
});
});
}
Using the
distinctUntilChanged along with debounce time in angular results better for your page. let's go throught this example so that you can understand distinctUntilChanged properly.
So in the above example , i added distinctUntilChanged operator along with debounce time , what actually was happened, if the user enter the same text again and again.
Summary
In conclusion, the debounceTime RxJS operator can be an incredibly useful tool when programming in Angular. It allows you to control the amount of time that passes before an action occurs, ensuring that your application isn't overwhelmed by unnecessary requests.
To use the debounceTime operator, you have to import it first and then wrap it around any code which triggers expensive or intensive operations. Make sure to test out different time intervals to find one that works best with your application.
Related articles:
0 Comments